Supported Runtimes
Write Scripts in Bash, Python, JavaScript and TypeScript
You can write scripts in all of the most popular scripting languages. Bash, Python, JavaScript and TypeScript are supported out-of-the-box.
Choose a runtime from the dropdown in the Editor tab.
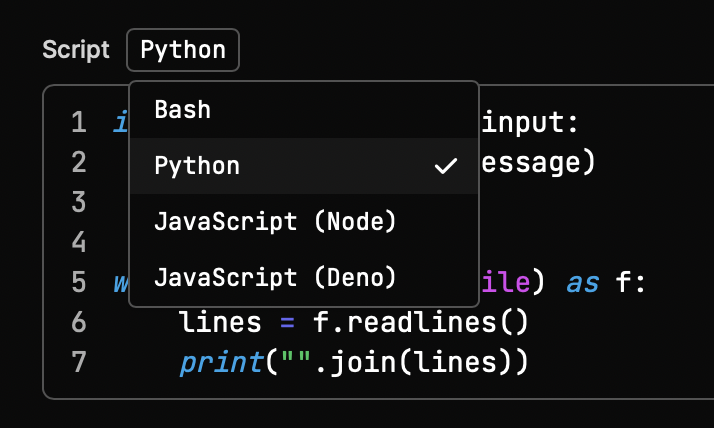
In order for your Fig script to be executed, the runtime must be installed and on your
PATH
. If the runtime binary is not installed, you will be prompted to install it.
Bash
Requires: bash
Object | Naming convention | Example |
---|---|---|
None | kebab-case | {{your-parameter-name}} |
Parameters passed to bash scripts are unquoted by default. Wrap inputs in single quotes to prevent them from being modified by the shell.
git commit -m '{{message}}'
Python
Requires: python3
Object | Naming convention | Example |
---|---|---|
fig.inputs | snake_case | fig.inputs.your_parameter_name |
When writing a script in Python, you can import libraries normally:
For instance, to manipulate PDFs using python, you can use the PyPDF2
library.
Run pip install PyPDF2
or pip3 install PyPDF2
to install the package, and then it will be accessible in your script.
import PyPDF2
pdf = open(fig.params.pdfpath, 'rb')
reader = PyPDF2.PdfFileReader(pdf)
# get first page of PDF
page = pdfReader.getPage(0)
# get the text from the first page
texts = page.extractText()
# print the text
print(texts)
JavaScript
Requires: node
or deno
Object | Naming convention | Example |
---|---|---|
fig.inputs | camelCase | fig.inputs.yourParameterName |
When writing a script in JavaScript, you can import libraries using ES6 syntax:
import fs from "node:fs"
let contents = fs.readFileSync(fig.inputs.filepath)
console.log(contents)
You can also import node packages that are installed globally.
For example, to use the cowsay package, install it with the -g flag:
npm install -g cowsay
import * as cowsay from "cowsay"
let output = cowsay.say({ text: fig.inputs.message });
console.log(output);
TypeScript
Requires: deno
Object | Naming convention | Example |
---|---|---|
fig.inputs | camelCase | fig.inputs.yourParameterName |
Missing a language?
Are we missing a language that your team uses to build scripts or internal CLI tools? Create a GitHub issue and we will add support.